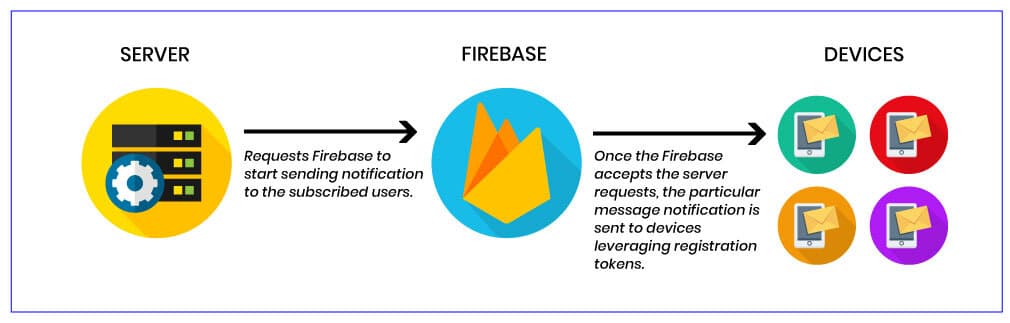
Setting Up Firebase Cloud Messaging (FCM) in Django Using Topic-Based Notifications
Introduction
Firebase Cloud Messaging (FCM) is a powerful cross-platform solution for sending notifications and messages. It allows you to deliver notifications to users across Android, iOS, and web apps. In this tutorial, we’ll walk you through setting up FCM in a Django project and using the fcm-django
package to send notifications to users subscribed to a topic when a new session is created.
We'll focus on sending notifications when a session is created in our university club management app. Notifications will be sent to users who are subscribed to a "Learners" topic.
Step 1: Setting Up Firebase Cloud Messaging on Firebase Console
Before we dive into the Django setup, you need to configure FCM in the Firebase Console.
1. Create a Firebase Project
- Go to the Firebase Console.
- Click on Add Project and follow the steps to create your project.
- Add Cloud Messaging app for your project
3. Generate the Private Key (Service Account)
To connect your Django app with Firebase, you need to download a private key JSON file.
- In the Firebase Console, go to Project Settings -> Service Accounts.
- Click on Generate New Private Key, and a JSON file will be downloaded.
- Place this JSON file in your Django project directory (e.g., in a folder named
config/
). - Add the following line to your
.env
file to set the path for the service account file:
GOOGLE_APPLICATION_CREDENTIALS=config/private-key-file-downloaded.json
This ensures that Firebase Admin SDK uses the service account credentials for authentication when sending notifications.
Step 2: Installing and Setting Up fcm-django
in Django
Next, we'll install the fcm-django
package and configure the Firebase settings.
1. Install fcm-django
Run the following command to install the package:
pip install fcm-django
2. Add the fcm-django
and Firebase Admin SDK to Installed Apps
Add 'fcm_django'
to your INSTALLED_APPS
in settings.py
:
INSTALLED_APPS = [
# Other apps
'fcm_django',
]
3. Configure Firebase Settings in settings.py
Now, we need to configure Firebase and FCM settings in the settings.py
file. First, initialize the Firebase app using the initialize_app
method, which will read the service account credentials from the .env
file.
from firebase_admin import initialize_app
# Initialize Firebase app
FIREBASE_APP = initialize_app()
# FCM Django Settings
FCM_DJANGO_SETTINGS = {
# Use the initialized Firebase app for FCM requests
"DEFAULT_FIREBASE_APP": None,
# Verbose name for the app
"APP_VERBOSE_NAME": "FCM Push Notifications App",
# Allow multiple devices for the same user
"ONE_DEVICE_PER_USER": False,
# Automatically delete inactive devices
"DELETE_INACTIVE_DEVICES": True,
}
Explanation of these settings:
DEFAULT_FIREBASE_APP
: Setting this toNone
ensures thatfcm-django
will use the default Firebase app initialized with theinitialize_app()
method.APP_VERBOSE_NAME
: A descriptive name for your FCM app.ONE_DEVICE_PER_USER
: Set toFalse
to allow users to have multiple devices registered for notifications.DELETE_INACTIVE_DEVICES
: If set toTrue
, inactive devices (those that return an error response) will be deleted from the system.
Step 3: Sending Notifications on Session Creation
Now that FCM is set up, we can send notifications when a new session is created. We’ll use Django signals to trigger notifications automatically whenever a session is added to the database.
Here’s how we can implement this feature:
Signal for Sending Notifications
We will use the post_save
signal to notify users when a new session is created. This notification will be sent to all users subscribed to the "Learners" topic.
from django.db.models.signals import post_save
from django.dispatch import receiver
from .models import Session
from firebase_admin import messaging
from notifications.models import Notification
from firebase_admin.exceptions import FirebaseError
from django.db import IntegrityError
@receiver(post_save, sender=Session)
def notify_users_on_new_session(sender, instance, created, **kwargs):
"""Notify users when a new session is created."""
if created:
title = "New Session Available!"
body = f"A new session '{instance.title}' has been scheduled. Check it out!"
try:
# Create an FCM notification for the topic 'Learners'
notification = messaging.Notification(title=title, body=body)
message = messaging.Message(notification=notification, topic="Learners")
# Send the FCM message to the topic
response = messaging.send(message)
# If the message is successfully sent, store it in the database
if response:
Notification.objects.create(title=title, body=body)
print("Successfully sent message:", response)
except FirebaseError as e:
# Handle Firebase errors (e.g., invalid token, API error, etc.)
print(f"Error sending FCM notification: {str(e)}")
except IntegrityError as e:
# Handle potential database errors (e.g., unique constraint issues)
print(f"Error saving notification to the database: {str(e)}")
How It Works:
- When a new session is created, the
post_save
signal is triggered. - We create a new FCM notification message with a title and body.
- The notification is sent to all users subscribed to the "Learners" topic via Firebase Cloud Messaging.
- If the notification is successfully sent, we save it in the
Notification
model.
Step 4: Testing the Setup
To test the notification functionality:
- Ensure your mobile app users are subscribed to the "Learners" topic (this should be done in the mobile app code).
- Create a new session in your Django app through the admin panel or API.
- Verify that the notification is sent to users subscribed to the topic.
Conclusion
In this tutorial, we’ve successfully integrated Firebase Cloud Messaging (FCM) with a Django application using the fcm-django
package. We configured the Firebase app, set up topic-based notifications, and sent a notification to users when a new session was created.
This setup provides a scalable way to deliver real-time notifications to groups of users, ensuring that important updates—such as session announcements—reach your app’s audience effectively.
By using topic-based messaging, you can easily organize users and target notifications to specific groups, making this approach ideal for apps with segmented user bases or event-driven updates.
Feel free to adapt this process for any notification scenarios in your Django app, whether it’s session creation, updates, or any other user events.